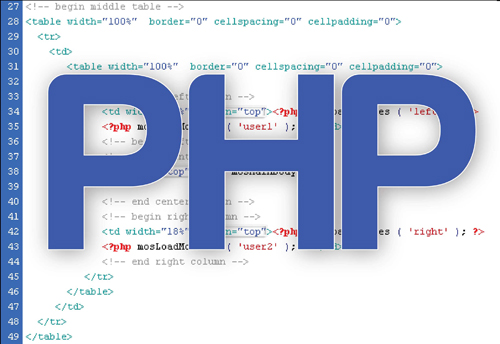
by Warren Gaebel | Apr 25, 2012
if ($a==1) { }elseif ($a==2) { }elseif ($a==3) { }elseif ($a==4) { ... }elseif ($a==23) { }elseif ($a==24) { }elseif ($a==25) { }else{ }
Option #2 is the same, except the keyword elseif is replaced by the two keywords else if:
if ($a==1) { }else if ($a==2) { }else if ($a==3) { }else if ($a==4) { ... }else if ($a==23) { }else if ($a==24) { }else if ($a==25) { }else{ }
Option #3 is a switch-case construct:
switch ($a) { case 1: break; case 2: break; case 3: break; case 4: break; ... case 23: break; case 24: break; case 25: break; default: }
In all cases, $a is initialized to 42 and the number of repetitions is set to 1,000,000 (what I usually use). The test results (in milliseconds) are:
Server # 1 ------------------------------ Option 1 Option 2 Option 3 -------- -------- -------- 1 2 1 1 2 2 2 2 2 2 1 2 1 2 1 2 1 2 1 2 1 2 1 2 1 2 1 1 2 1 Server # 2 ------------------------------ Option 1 Option 2 Option 3 -------- -------- -------- 0 1 0 0 1 1 0 1 0 1 0 1 1 0 1 0 1 0 1 1 0 1 1 0 0 1 0 0 1 1
Option #2 (if-elseif-else) is the slowest more than half the time. The other two are about equal.
However, are these results statistically significant? I don’t have the time or the expertise to do the calculations, but I remember from my statistics classes that anything with less than 50 trials is suspect. Let’s do 10 more trials and see if we get the same results.
Server # 1 ------------------------------ Option 1 Option 2 Option 3 -------- -------- -------- 2 2 1 1 1 2 2 1 2 2 2 2 1 2 2 1 2 1 2 2 2 2 1 2 1 2 1 2 1 2 Server # 2 ------------------------------ Option 1 Option 2 Option 3 -------- -------- -------- 1 0 1 1 1 0 0 1 1 1 0 1 0 1 0 0 1 0 1 0 1 1 0 1 1 1 0 0 1 0
These data lead to a different conclusion. Here there is no significant difference between the three options.
I’m stopping here because the tests are showing a maximum of 1 millisecond difference between the options. Even if we were to conclude that one option is faster than the other, one millisecond for a million tests is just not enough of a difference to matter.
After completing this article, I felt guilty about using nulls within the then and else clauses, so I reran the tests with $b = rand() inside every then, else, and case clause. The results were almost identical, and the conclusion was the same.
I get a gold star for guessing correctly on this one.
for ($i = 0; $i < $numReps; $i++) {$x = rand();}
Option #2 is the same, except the postincrement of the loop control variable is changed to a preincrement:
for ($i = 0; $i < $numReps; ++$i) {$x = rand();}
Option #3 combines the increment and test into one, which eliminates the increment section of the for. Note that option #3 is not equivalent to the first two options because the loop control variable ($i) increments in the first two options, but in the third option it decrements.
for ($i = $numReps; $i--;) {$x = rand();}
As always, the number of repetitions is set to 1,000,000. The results (in milliseconds) are:
Server # 1 ------------------------------ Option 1 Option 2 Option 3 -------- -------- -------- 0 1 0 1 0 1 0 1 0 0 1 0 0 0 0 1 0 0 0 1 0 1 0 0 0 1 0 0 0 1 Server # 2 ------------------------------ Option 1 Option 2 Option 3 -------- -------- -------- 0 0 0 1 0 0 0 1 0 0 0 1 0 0 0 0 0 0 0 0 1 1 0 0 0 0 0 0 0 0
With all those zeroes, one might wonder why we’re spending any time at all on this. According to these results, the biggest difference we can hope for is one millisecond per million tests.
We must be careful with our performance tests. I didn’t use empty bodies inside the loops because an optimizer could easily throw away the iteration construct and replace it with a simple assignment to $i.
Another gold star to add to my collection.
for ($i = 0; $i < $numReps; ++$i) { $x = rand(); }
Option #2 is a while loop:
$i = 0; while ($i < $numReps) { $x = rand(); ++$i; }
Option #3 is a do-while loop. Note that option #3 is not equivalent to the other two because it forces the first iteration even if the condition is false. This doesn’t matter in the test we are conducting today, but it may matter in real-world code.
$i = 0; do { $x = rand(); ++$i; } while ($i < $numReps);
As always, the number of repetitions is set to 1,000,000. The results (in milliseconds) are:
Server # 1 ------------------------------ Option 1 Option 2 Option 3 -------- -------- -------- 0 1 0 1 0 0 0 1 0 0 1 0 0 0 1 0 0 1 1 0 0 1 0 1 0 1 0 0 0 1 Server # 2 ------------------------------ Option 1 Option 2 Option 3 -------- -------- -------- 0 0 1 0 0 0 1 0 0 0 0 0 0 1 0 0 1 0 0 0 0 0 0 1 1 0 0 0 0 0
And once again, we’re seeing such small numbers that it hardly seems worth our while. Even if we did consider these numbers significant, the test results show no real difference between the three options.
So I go away with three gold stars today. But I can’t really take that much credit – it was pretty obvious.
Category: Website Performance | Tagged No Comments.